Increasing efficiency with Templates
Increase your coding efficiency by creating your own code templates.
While I was preparing for a timed coding interview, increasing my coding speed becomes one of the topic I had to do research on. Right then, I found out about using Live Templates to an extent. I knew it existed but I never had really used before that much. I started making a habit of using it, and I felt that a lot of the repetitive code has been less painful to write. Live Template is similar to code completion, if you have ever generated a method from an lint error, you will be familiar with something like this
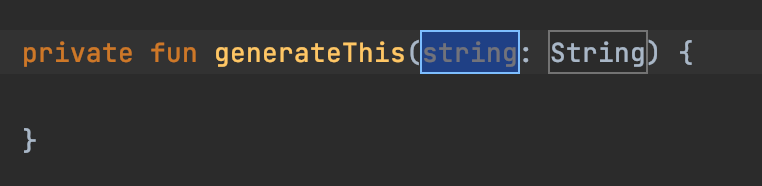
Live Template is similar in that it generates a piece of code with some variables/placeholders in it. The cursor will be automatically placed on the placeholders for you to edit, one after another (Press Tab or Enter to switch to next placeholder). If you're on your IntelliJ right now as you read this, try typing inn
in a Kotlin class file and select the first result listed in the auto complete (Control+Space to bring it up on mac and Alt+Space on Windows). You will see that a function similar to this is generated
if (someNullableVariable != null) {
}
someNullableVariable
Doesn't that feel convenient? You don't have to type the whole if block. Typing 3 letters, inn
will generate it for you. There's a couple of them already bundled in IntelliJ by default, you can check the rest out in Preferences => Editor => Live Templates. But let's not stop there, we can create a new one for Kotlin, ourselves.
If you have been developing Android for a while, you might be familiar with the concept of MutableLiveData
and LiveData
. For those of you not familiar with it, you can think of it as MutableList
and List
. One is mutable, and the other one is immutable. The best practice is that our API should not expose mutable ones to be consumed, because if we do, the consumer could mutate the behavior into something not intended by our APIs. Hence, if we follow the best practice, our code should looks something like this
class ViewModel {
private val _nameLiveData = MutableLiveData<String>()
val nameLiveData : LiveData<String>() get() = _nameLiveData
_nameLiveData.postValue("Aung Kyaw Paing")
}
val viewModel = ViewModel()
viewModel.nameLiveData.postValue("Vincent") //Can't do this
This way, our code are nicely encapsulated within the ViewModel
and whoever consume our API cannot mutate the value that we are emitting. This is all great but it's really annoying to write the same two variable declaration lines over and over again every time you need a MutableLiveData
. Let's make this task easier on us by writing our own custom live template.
First, let's go back to the Live Template preferences again (Preferences => Editor => Live Templates). Click on the "+" button at the top right of the panel. Let's give the abbreviation, vmmld
,which would stands for ViewModel Mutable Live Data. For description, you can type "MutableLiveData declaration inside ViewModel", or anything that you see fits.
The code we want to be templated would be these two lines:
private val _nameLiveData = MutableLiveData<String>()
val nameLiveData : LiveData<String>() get() = _nameLiveData
We can see that there are two variables. First is name; where for MutableLiveData
we append _
as prefix to differentiate easily. Second one would be the variable type of MutableLiveData
and LiveData
. In short, we can reformat into these lines:
private val _{NAME} = MutableLiveData<{TYPE}>()
val {NAME} : LiveData<{TYPE}>() get() = _{NAME}
We will need to translate this to the format Live Template use. In Live Template, you can declare a variable
by writing the name of the variable within two $
symbol. For example, our name variable would be defined as $NAME$
. If we convert the above code onto our code template, we'd get the following:
private val _$NAME$ = MutableLiveData<$TYPE$>()
val $NAME$ : LiveData<$TYPE$> get() = _$NAME$
After this, we need to define where this abbreviation can be used. For this case, we will set the Applicable Context to Kotlin classes. Simply click the Change beside No Applicable Context, which you will find underneath the template text. And select Kotlin => Class. Finally, click Apply and close the menu, and we can test it out by typing out vvmld
in a Kotlin class and it will generate the two lines for you. We can quickly enter the name and type without having to write the two lines all the time.
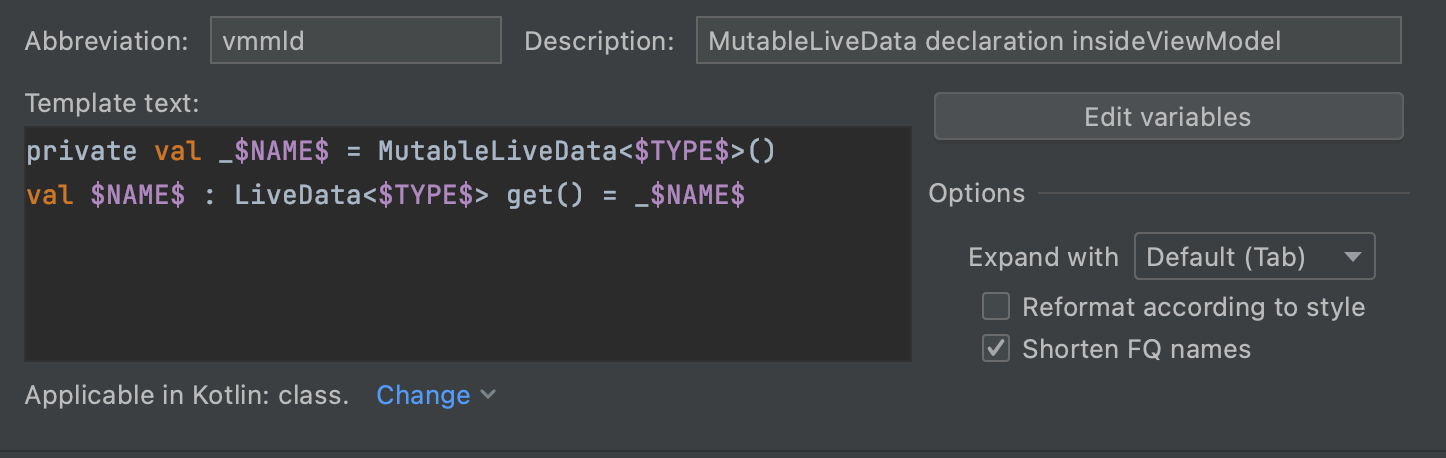
It doesn't end there. We can also use functions inside Live Template. If you click on "Edit Variable" in the template menu. It will shows you a table with some columns. You can define an expression, for example, if we want to format a current date into the template, we can use date()
function. Plus, we can even specify a default value that will automatically be filled for you. You can find out more about the predefined functions here. With this knowledge, I hope you come up with some templates that will help you in writing repetitive code over and over again. Cheers.